Get Started
This guide is intended for publishers who want to monetize a Unity app.
Integrating the Google Mobile Ads Unity plugin into an app, which you will do here, is the first step toward displaying AdPumb ads and earning revenue. Once the integration is complete, you can choose an ad format (such as native or rewarded video) to get detailed implementation steps.
Prerequisite
You need to register with us before starting the integration. You can drop a mail to sales@adpumb.com
Steps
1. Enable custom Manifest and gradle.
Edit > Project Settings > Player then click on Android > publishing settings to add admob publisher id and gradle library dependences
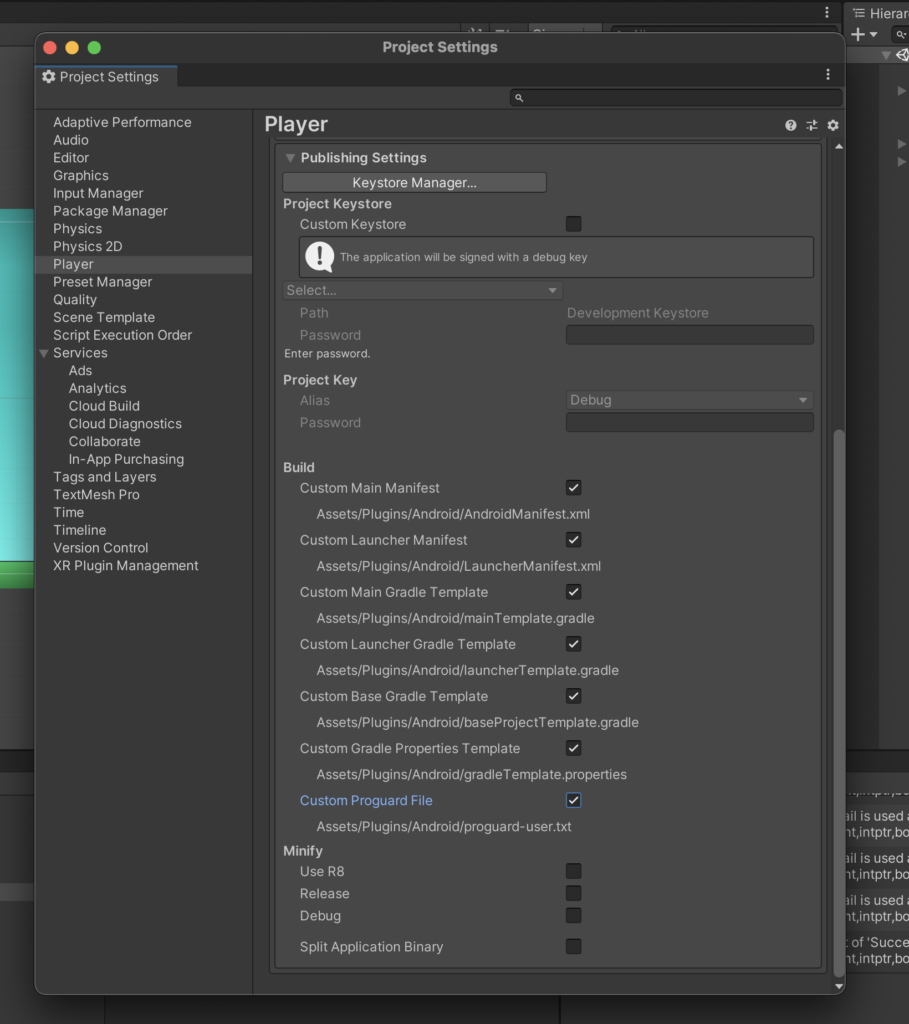
- Add your admob publisher id to the android-manifest.xml ( located in Assets > Plugins > Android ) of your app: Adpump doesn’t use your admob adunits, however adpump uses the underlying admob APIs for which publisher id is mandatory.
- Add library dependency: it is needed to add the repository details and dependency to your gradle script.
Add repository to you baseProjectTemplate.gradle file ( located in Assets > Plugins > Android ) of your app
repositories{
maven {
url 'https://maven.adpumb.com/repository/adpumb/'
}
}
Add dependency to you mainTemplate.gradle file ( located in Assets > Plugins > Android ) of your app
dependencies {
implementation 'com.adpumb:bidmachine:2.4.9'
*********************
Also needed to activate AndroidX and enable Jetifier in gradleTemplate.properties file and enable multiDex
android.useAndroidX=true
android.enableJetifier=true
in launcherTemplate.gradle
defaultConfig {
.....
multiDexEnabled true
.....
}
- Adding config key to AndroidManifestOn AndroidManifest.xml add meta data with name ‘com.adpumb.config.key’ values given by us.
<meta-data
android:name="com.adpumb.config.key"
android:value="adpumb-key-goes-here" />
- Create a Folder AdPumb in Assets > Plugins then copy contents from With THIS FILE to a C# Script file AdPumbPlugin.cs .
- Create placement: Adpump is designed on the concept of placement rather than adunit. A placement is a predefined action sequence which ends up in showing an Ad.
AdPlacementBuilder placementObject1 = AdPlacementBuilder.Interstitial()
.name("ad_placement_name")
.showLoaderTillAdIsReady(true)
.loaderTimeOutInSeconds(5)
.frequencyCapInSeconds(15);
DisplayManager.Instance.showAd(placementObject1);
In this example if the ad is not ready within 5 seconds then the loader will be removed. However if the ad is already loaded or it got loaded while the loader is shown, then ad loader will be hidden and ad will be shown to user.
- For a particular placement you need to create only one placement object, which can be used to show multiple ads.
For example:
AdPlacementBuilder placementObject2 = AdPlacementBuilder.Interstitial()
.name("2nd_ad_placement_name");
// somewhere in the class calling an ad
DisplayManager.Instance.showAd(placementObject2);
// somewhere in the class calling another ad
DisplayManager.Instance.showAd(placementObject2);
- Callbacks: You can register callbacks to the placement.
AdPlacementBuilder placementObject3 = AdPlacementBuilder.Interstitial()
.name("3rd_ad_placement_name")
.showLoaderTillAdIsReady(true)
.loaderTimeOutInSeconds(5)
.frequencyCapInSeconds(15)
.onAdCompletion( this.onAdCompletion );
DisplayManager.Instance.showAd(placementObject3);
.........
public void onAdCompletion(bool success,bool isAdFailed){
if(isAdFailed){
// ad failed to load
} else if(isSuccess){
// watched ad
} else {
// Didn't watched the ad
}
}
- Customising loader : You can customize the loader using the loader settings for each placement
LoaderSettings loader = new LoaderSettings();
loader.setLogoResID(); // this will set loader logo to your app logo
AdPlacementBuilder placementObject4 = AdPlacementBuilder.Interstitial()
.name("4th_ad_placement_name")
.showLoaderTillAdIsReady(true)
.loaderTimeOutInSeconds(5)
.frequencyCapInSeconds(15)
.loaderUISetting(loader)
.onAdCompletion( this.onAdCompletion ) ;
DisplayManager.Instance.showAd(placementObject4);
- Analytics : event with impression info
ExternalAnalytics.Instance.onEvent(this.AdPumbAnalyticsEvent);
void AdPumbAnalyticsEvent(string placement,float eCPM){
Debug.Log(" placement : "+placement+" - eCPM : "+eCPM );
}
9. Deferent types of ads
AdPlacementBuilder placement2 = AdPlacementBuilder.Interstitial()
AdPlacementBuilder placement3 = AdPlacementBuilder.Rewarded()
AdPlacementBuilder placement4 = AdPlacementBuilder.AppOpenInterstitial()
AdPlacementBuilder placement5 = AdPlacementBuilder.RewardedInterstitial()